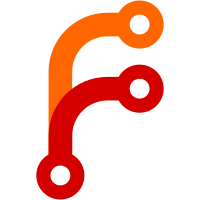
Change the notifications subpages to be distinct route handlers with actual paths rather than query parameters. They already were a massive conditional chain with almost no common logic. Hard wrap some of the more egregious query lines. Use less duplicated code for shadowban exclusion. Only major functionality change which is somewhat related to #476 is to sort subtrees by Comment.id DESC. Otherwise, upstream is substantially the same as TheMotte. Given that upstream didn't experience #476, I think the issue may have been resolved by prior changes to filtering / comment visibility & moderation.
64 lines
2.1 KiB
Python
64 lines
2.1 KiB
Python
import sys
|
|
|
|
import gevent
|
|
from pusher_push_notifications import PushNotifications
|
|
from sqlalchemy.orm import Session
|
|
|
|
from files.__main__ import db_session, service
|
|
from files.classes.leaderboard import (GivenUpvotesLeaderboard,
|
|
LeaderboardMeta,
|
|
ReceivedDownvotesLeaderboard)
|
|
from files.helpers.assetcache import assetcache_path
|
|
from files.helpers.config.environment import (ENABLE_SERVICES, PUSHER_ID,
|
|
PUSHER_KEY, SITE_FULL, SITE_ID)
|
|
|
|
if service.enable_services and ENABLE_SERVICES and PUSHER_ID != 'blahblahblah':
|
|
beams_client = PushNotifications(instance_id=PUSHER_ID, secret_key=PUSHER_KEY)
|
|
else:
|
|
beams_client = None
|
|
|
|
def pusher_thread2(interests, notifbody, username):
|
|
if not beams_client: return
|
|
beams_client.publish_to_interests(
|
|
interests=[interests],
|
|
publish_body={
|
|
'web': {
|
|
'notification': {
|
|
'title': f'New message from @{username}',
|
|
'body': notifbody,
|
|
'deep_link': f'{SITE_FULL}/notifications/messages',
|
|
'icon': SITE_FULL + assetcache_path(f'images/{SITE_ID}/icon.webp'),
|
|
}
|
|
},
|
|
'fcm': {
|
|
'notification': {
|
|
'title': f'New message from @{username}',
|
|
'body': notifbody,
|
|
},
|
|
'data': {
|
|
'url': '/notifications/messages',
|
|
}
|
|
}
|
|
},
|
|
)
|
|
sys.stdout.flush()
|
|
|
|
_lb_received_downvotes_meta = LeaderboardMeta("Downvotes", "received downvotes", "received-downvotes", "downvotes", "downvoted")
|
|
_lb_given_upvotes_meta = LeaderboardMeta("Upvotes", "given upvotes", "given-upvotes", "upvotes", "upvoting")
|
|
|
|
lb_downvotes_received: ReceivedDownvotesLeaderboard | None = None
|
|
lb_upvotes_given: GivenUpvotesLeaderboard | None = None
|
|
|
|
def leaderboard_thread():
|
|
global lb_downvotes_received, lb_upvotes_given
|
|
|
|
db: Session = db_session()
|
|
|
|
lb_downvotes_received = ReceivedDownvotesLeaderboard(_lb_received_downvotes_meta, db)
|
|
lb_upvotes_given = GivenUpvotesLeaderboard(_lb_given_upvotes_meta, db)
|
|
|
|
db.close()
|
|
sys.stdout.flush()
|
|
|
|
if service.enable_services and ENABLE_SERVICES:
|
|
gevent.spawn(leaderboard_thread())
|